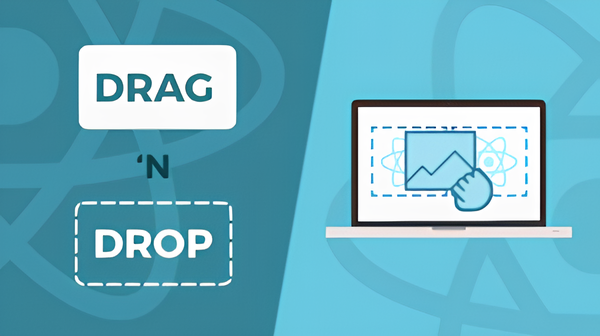
React 使用 React Dnd 实现列表拖拽排序
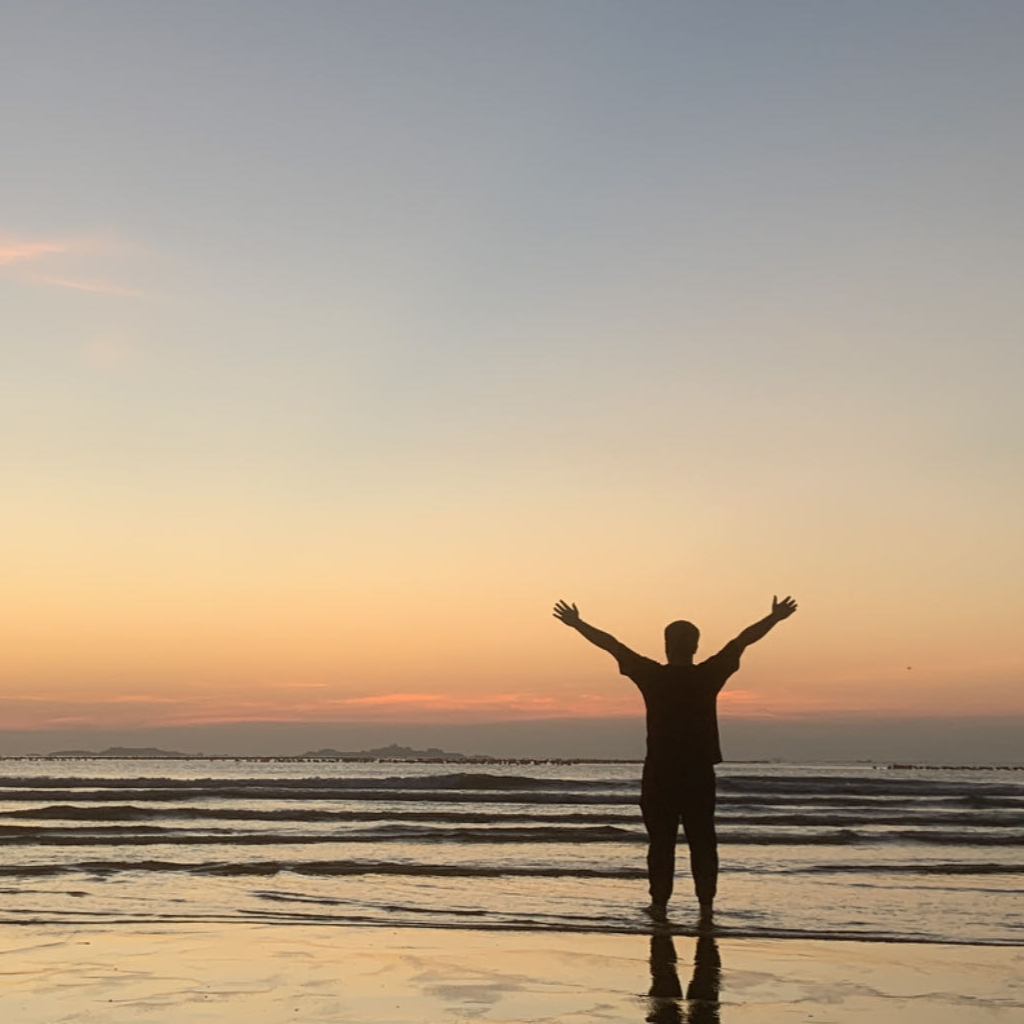
- 前言
拖拽排序功能在现代的网页应用中非常常见,它提供了一种直观、灵活的方式来让用户自定义内容的顺序。React Dnd 是基于 HTML5 的拖放 API 构建的,它能够让你轻松地在 React 应用中添加拖拽功能。
本文介绍如何利用 React Dnd(Drag and Drop)这个强大的库来实现一个简易的列表拖拽排序功能。
实现步骤
一、安装 React Dnd
首先,我们需要在项目中安装 React Dnd 及其 HTML5 后端库:
bashnpm install react-dnd react-dnd-html5-backend
或者,如果你使用 yarn
作为包管理器:
bashyarn add react-dnd react-dnd-html5-backend
二、实现拖拽
接下来,我们将逐步实现拖拽排序列表功能。
1. 设置 DndProvider
为了使 React Dnd 能够在你的应用中运作,你需要在应用的最顶层包裹上 DndProvider
组件,并传递给它 HTML5 后端。
jsximport { DndProvider } from 'react-dnd'; import { HTML5Backend } from 'react-dnd-html5-backend'; function App() { return ( <DndProvider backend={HTML5Backend}> {/* 你的其他组件将在这里 */} </DndProvider> ); }
2. 创建可拖拽的列表项组件
每个列表项都需要变成一个可拖拽的组件。我们使用 useDrag
钩子来定义拖拽源。
jsximport { useDrag } from 'react-dnd'; const ItemTypes = { LIST_ITEM: 'listItem' }; function DraggableListItem({ id, text, index, moveListItem }) { const [{ isDragging }, drag] = useDrag({ type: ItemTypes.LIST_ITEM, item: { id, index }, collect: (monitor) => ({ isDragging: monitor.isDragging() }) }); const opacity = isDragging ? 0.5 : 1; return ( <div ref={drag} style={{ opacity }}> {text} </div> ); }
3. 创建可放置的列表组件
列表本身需要能够接收拖拽的组件。这是通过 useDrop
钩子来实现的。
jsximport { useDrop } from 'react-dnd'; function DroppableList({ items, moveListItem }) { const [, drop] = useDrop({ accept: ItemTypes.LIST_ITEM, hover(item, monitor) { const dragIndex = item.index; const hoverIndex = items.findIndex((i) => i.id === item.id); if (dragIndex === hoverIndex) { return; } const hoverBoundingRect = monitor.getClientOffset(); // ... // 交换位置的逻辑 moveListItem(dragIndex, hoverIndex); item.index = hoverIndex; } }); return ( <div ref={drop}> {items.map((item, index) => ( <DraggableListItem key={item.id} index={index} id={item.id} text={item.text} moveListItem={moveListItem} /> ))} </div> ); }
4. 状态管理与位置交换逻辑
现在需要在父组件中实现状态管理以及拖拽过程中列表项位置交换的逻辑。
jsx// 首先,我们设定列表的初始状态 import React, { useState, useCallback } from 'react'; import { DndProvider } from 'react-dnd'; import { HTML5Backend } from 'react-dnd-html5-backend'; // ... 其他导入项和组件定义 function App() { const [items, setItems] = useState([ { id: 1, text: 'Item 1' }, { id: 2, text: 'Item 2' }, { id: 3, text: 'Item 3' }, // ... 更多列表项 ]); // 定义一个函数来处理位置交换逻辑 const moveListItem = useCallback((dragIndex, hoverIndex) => { // 复制当前的 items 数组 const newItems = [...items]; // 取出被拖拽的元素 const [draggedItem] = newItems.splice(dragIndex, 1); // 将被拖拽的元素插入到新的位置 newItems.splice(hoverIndex, 0, draggedItem); // 更新状态 setItems(newItems); }, [items]); return ( <DndProvider backend={HTML5Backend}> {/* 将 items 和 moveListItem 传递给可放置的列表组件 */} <DroppableList items={items} moveListItem={moveListItem} /> </DndProvider> ); }
现在的应用已经有了拖拽排序列表的基本功能。用户可以通过拖拽列表项来重新排序它们。
5. 完善 hover 方法和样式
在 useDrop
hook 的 hover
方法中,我们还需要添加更多的逻辑来处理当拖动项悬停在另一个项上方时的情况。我们也可以加入一些基本样式来改善用户体验。
jsx// ... 其他导入项和组件定义 function DroppableList({ items, moveListItem }) { const [, drop] = useDrop({ accept: ItemTypes.LIST_ITEM, hover(item, monitor) { const dragIndex = item.index; const hoverIndex = items.findIndex((i) => i.id === item.id); // 如果位置相同,则不做处理 if (dragIndex === hoverIndex) { return; } // 确定屏幕上的悬停区域 const hoverBoundingRect = monitor.getClientOffset(); // ... 这里可以加入更多的计算逻辑,以精确判断什么时候触发排序动作 // 交换位置的逻辑 moveListItem(dragIndex, hoverIndex); // 更新拖拽项的索引值,因为排序操作已经发生 item.index = hoverIndex; } }); // 添加一些基本样式 const style = { backgroundColor: '#fff', padding: '10px', margin: '10px', border: '1px solid #ddd', borderRadius: '4px' }; return ( <div ref={drop} style={style}> {items.map((item, index) => ( <DraggableListItem key={item.id} index={index} id={item.id} text={item.text} moveListItem={moveListItem} /> ))} </div> ); } // ... App 组件和其他代码
通过上面的步骤,你已经可以创建一个基础的拖拽排序列表。不过,在真实的应用中,你可能需要处理更复杂的情况和细节,例如处理大量数据、优化性能、增加动画效果等。React Dnd 提供了丰富的 API 和钩子函数来帮助你完成这些高级功能。