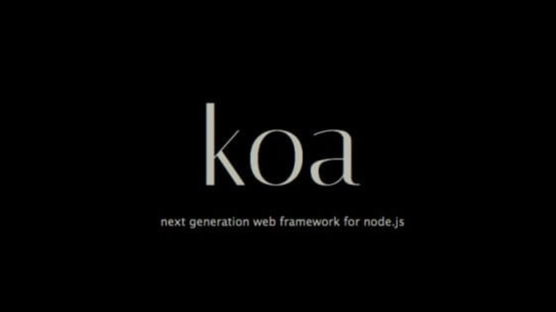
前端快速入门Koa.js
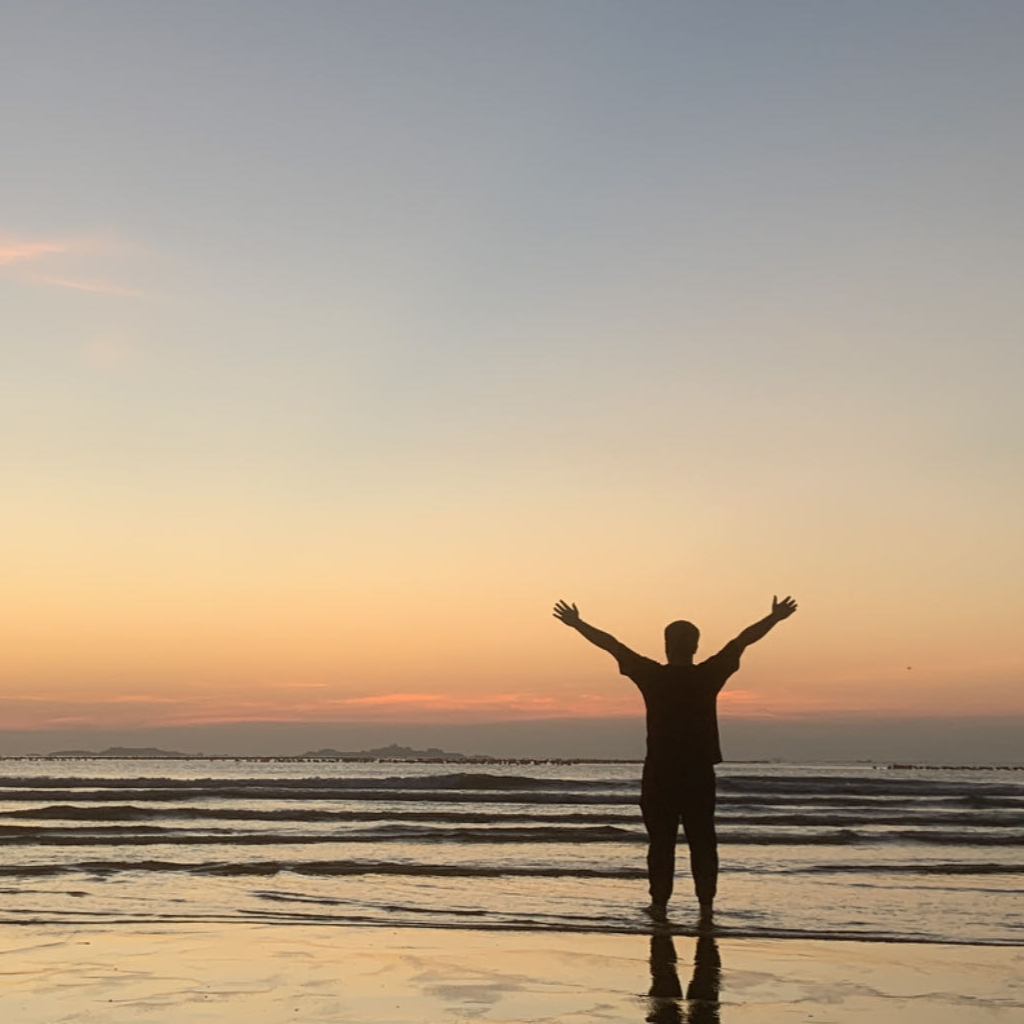
前言
Nodejs 提供了 http 能力,我们通过如下代码可以快速创建一个http server服务
javascriptconst http = require('http'); http .createServer((req, res) => { res.write('hello\n'); res.end(); }) .listen(3000);
使用nodejs提供的原生能力启动一个http server并不麻烦,但是拓展额外的能力就比较麻烦,比如支持路由router,静态资源,页面模板等。
插件机制是目前非常流行的拓展能力的设计方式,Koa框架是基于插件机制封装出来的一个Node HTTP框架,我将简单记录一下Koa的插件机制以及丰富的插件。
快速启动
javascript// app.js const Koa = require('koa'); const app = new Koa(); app.use(async ctx => { ctx.body = 'Hello World'; }); app.listen(9999,()=>{ console.log('App start on port: 9999') });
json// package.json { "scripts": { "dev": "node app.js" } }
中间件Middleware(洋葱模型)
通过 Koa.use 将中间件注册到 koa应用中,中间件可以注册N个
我们可以通过插件机制个性化功能,提供给别人复用
中间件格式 async (ctx,next)=>{}
- ctx => context对象,包含request,response对象,我们可以通过ctx来处理自己的业务需求
- next ,promise对象,可以通过await next(),让程序执行下一个中间件,执行完后再执行当前中间件next下面的逻辑
javascriptconst Koa = require('koa'); const app = new Koa(); app.use(async(ctx,next)=>{ console.log('middleware one in') await next(); console.log('middleware one out') }) app.use(async(ctx,next)=>{ console.log('middleware two in') await next(); console.log('middleware two out') }) app.use(async(ctx,next)=>{ console.log('middleware three in') await next(); console.log('middleware three out') }) app.listen(9999, () => { console.log("app started! port:9999"); });
运行结果
javascriptmiddleware one in middleware two in middleware three in middleware three out middleware two out middleware one out
中间件运行过程
常用中间件
Koa Router
作为一个HTTP server,处理不同path的请求是最常见的问题,koa router就是专门处理路由分发的中间件
主程序
javascriptconst Koa = require('koa'); const Router = require('koa-router'); const app = new Koa(); const router = new Router(); router.get('/',async(ctx)=>{ ctx.type = 'html'; ctx.body = '<h1>hello world!</h1>'; }) app.use(router.routes()); app.use(router.allowedMethods()) app.listen(9999, () => { console.log("app started! port:9999"); });
运行结果
属性简介
Router实例上提供了http的多种请求方式 get
post
put
delete
等等。router的请求方法会接受两个参数,第一个参数是匹配的请求的路径 path,第二个参数是处理逻辑的函数。
Router的 routes,allowedMethods方法返回参数,是koa实例接受中间件格式。
Koa View
页面渲染器,支持多种成熟的模版解析引擎。
shellnpm add koa-view ejs
主程序代码
javascript//app.js const Koa = require('koa'); const Router = require('koa-router'); const views = require('koa-views'); const app = new Koa(); const router = new Router(); app.use(views(__dirname + '/views', { map: { html: 'ejs' } })) router.get('/home',async(ctx)=>{ await ctx.render('home',{words:'欢迎你'}) }) app.use(router.routes()); app.use(router.allowedMethods()) app.listen(9999, () => { console.log("app started! port:9999"); });
html模版
html// views/home.html <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>乐闻世界</title> </head> <body> 乐闻世界<%= words %> </body> </html>
运行效果
Koa Static
koa 支持静态资源的请求
相关依赖
shellyarn add koa-static
主程序
javascriptconst Koa = require('koa'); const static = require('koa-static'); const app = new Koa(); app.use(static(__dirname + '/statics')) app.listen(9999, () => { console.log("app started! port:9999"); });
运行效果